3.5.2.1.42. SourceCodeEditor
SourceCodeEditor
is designed to display and enter source code. It is a multi-line text area featured with code highlighting and optional print margin and gutter with line numbers.
XML-name of the component: sourceCodeEditor
.
SourceCodeEditor
is implemented for Web Client.
Basically, SourceCodeEditor
mostly replicates the functionality of the TextField component and has the following specific attributes:
-
if
handleTabKey
istrue
, the Tab button on the keyboard is handled to indent lines, whenfalse
, it is used to advance the cursor or focus to the next tab stop. This attribute should be set when the screen is initialized and will not work if changed at a runtime.
All following properties can be easily changed at a runtime:
-
highlightActiveLine
is used to highlight the line the caret is on.
-
mode
provides the list of languages supported for the syntax highlight. This list is defined in theMode
enumeration of theSourceCodeEditor
interface and includes the following languages: Java, HTML, XML, Groovy, SQL, JavaScript, Properties, and Text with no highlight.
-
printMargin
attribute sets if the printing edge line should be displayed or hidden.
-
showGutter
is used to hide or show the left gutter with line numbers.
Below is an example of SourceCodeEditor
component adjustable at a runtime.
XML-descriptor:
<hbox spacing="true">
<checkBox id="highlightActiveLineCheck" align="BOTTOM_LEFT" caption="Highlight Active Line"/>
<checkBox id="printMarginCheck" align="BOTTOM_LEFT" caption="Print Margin"/>
<checkBox id="showGutterCheck" align="BOTTOM_LEFT" caption="Show Gutter"/>
<lookupField id="modeField" align="BOTTOM_LEFT" caption="Mode" required="true"/>
</hbox>
<sourceCodeEditor id="simpleCodeEditor" width="100%"/>
The controller:
@Inject
private CheckBox highlightActiveLineCheck;
@Inject
private LookupField<HighlightMode> modeField;
@Inject
private CheckBox printMarginCheck;
@Inject
private CheckBox showGutterCheck;
@Inject
private SourceCodeEditor simpleCodeEditor;
@Subscribe
protected void onInit(InitEvent event) {
highlightActiveLineCheck.setValue(simpleCodeEditor.isHighlightActiveLine());
highlightActiveLineCheck.addValueChangeListener(e ->
simpleCodeEditor.setHighlightActiveLine(Boolean.TRUE.equals(e.getValue())));
printMarginCheck.setValue(simpleCodeEditor.isShowPrintMargin());
printMarginCheck.addValueChangeListener(e ->
simpleCodeEditor.setShowPrintMargin(Boolean.TRUE.equals(e.getValue())));
showGutterCheck.setValue(simpleCodeEditor.isShowGutter());
showGutterCheck.addValueChangeListener(e ->
simpleCodeEditor.setShowGutter(Boolean.TRUE.equals(e.getValue())));
Map<String, HighlightMode> modes = new HashMap<>();
for (HighlightMode mode : SourceCodeEditor.Mode.values()) {
modes.put(mode.toString(), mode);
}
modeField.setOptionsMap(modes);
modeField.setValue(HighlightMode.TEXT);
modeField.addValueChangeListener(e ->
simpleCodeEditor.setMode(e.getValue()));
}
The result will be:
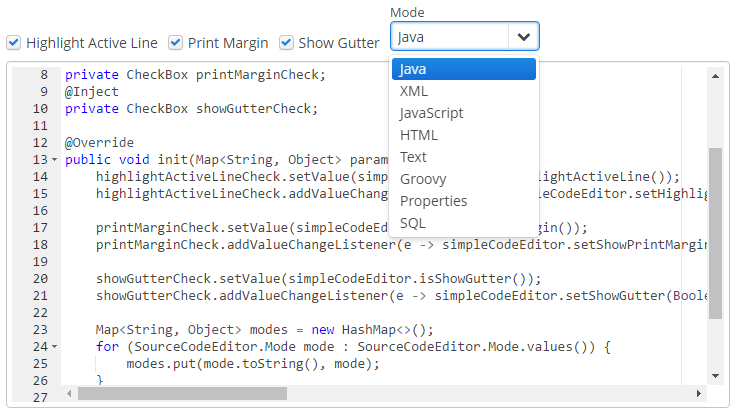
SourceCodeEditor
can also support code autocomplete provided by the Suggester
class. To activate word completion, the setSuggester
method should be invoked, for example:
@Inject
protected DataGrid<User> usersGrid;
@Inject
private SourceCodeEditor suggesterCodeEditor;
@Inject
private CollectionContainer<User> usersDc;
@Inject
private CollectionLoader<User> usersDl;
@Subscribe
protected void onInit(InitEvent event) {
suggesterCodeEditor.setSuggester((source, text, cursorPosition) -> {
List<Suggestion> suggestions = new ArrayList<>();
usersDl.load();
for (User user : usersDc.getItems()) {
suggestions.add(new Suggestion(source, user.getLogin(), user.getName(), null, -1, -1));
}
return suggestions;
});
}
The result:
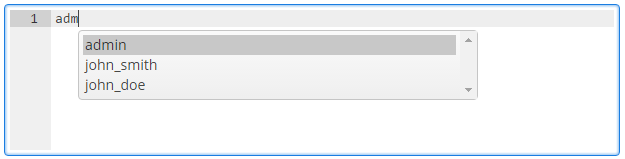
Ctrl+Space or pressed dot invokes suggestions. To disable code autocomplete after pressing a dot, set the suggestOnDot
attribute in false
. The default value of this option is true
.
<sourceCodeEditor id="simpleCodeEditor" width="100%" suggestOnDot="false"/>
The appearance of the SourceCodeEditor
component can be customized using SCSS variables with $cuba-sourcecodeeditor-*
prefix. You can change these variables in the visual editor after creating a theme extension or a custom theme.
- Attributes of sourceCodeEditor
-
align - caption - captionAsHtml - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - dataContainer - description - descriptionAsHtml - editable - enable - box.expandRatio - handleTabKey - height - htmlSanitizerEnabled - highlightActiveLine - icon - id - mode - printMargin - property - required - requiredMessage - rowspan - suggestOnDot - showGutter - stylename - tabIndex - visible - width
- API