3.5.2.1.2. BrowserFrame
A BrowserFrame
is designed to display embedded web pages. It is the equivalent of the HTML iframe
element.
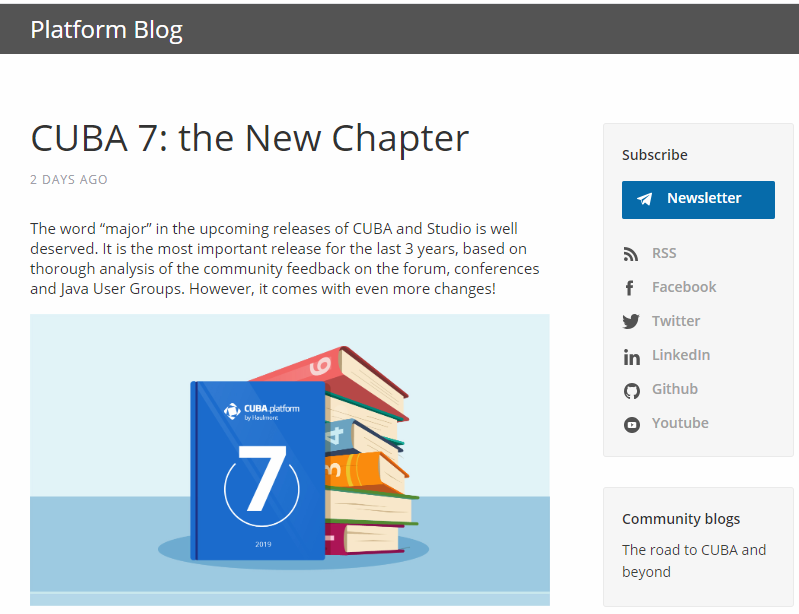
Component’s XML-name: browserFrame
An example of component definition in an XML-descriptor of a screen:
<browserFrame id="browserFrame"
height="280px"
width="600px"
align="MIDDLE_CENTER">
<url url="https://www.cuba-platform.com/blog/cuba-7-the-new-chapter"/>
</browserFrame>
Similarly to the Image component, the BrowserFrame
component can also display images from different resources. You can set the resource type declaratively using the browserFrame
elements listed below:
-
classpath
- a resource in the classpath.<browserFrame> <classpath path="com/company/sample/web/screens/myPic.jpg"/> </browserFrame>
-
file
- a resource in the file system.<browserFrame> <file path="D:\sample\modules\web\web\VAADIN\images\myImage.jpg"/> </browserFrame>
-
relativePath
- a resource in the application directory.<browserFrame> <relativePath path="VAADIN/images/myImage.jpg"/> </browserFrame>
-
theme
- a theme resource, for example:<browserFrame> <theme path="../halo/com.company.demo/myPic.jpg"/> </browserFrame>
-
url
- a resource which can be loaded from the given URL.<browserFrame> <url url="http://www.foobar2000.org/"/> </browserFrame>
browserFrame
attributes:
-
The
allow
attribute specifies Feature Policy for the component. The value of the attribute should be a space-separated list of allowed features:-
autoplay
– controls whether the current document is allowed to autoplay media requested through the interface. -
camera
– controls whether the current document is allowed to use video input devices. -
document-domain
– controls whether the current document is allowed to setdocument.domain
. -
encrypted-media
– controls whether the current document is allowed to use the Encrypted Media Extensions API (EME). -
fullscreen
– controls whether the current document is allowed to useElement.requestFullScreen()
. -
geolocation
– controls whether the current document is allowed to use the Geolocation Interface. -
microphone
– controls whether the current document is allowed to use audio input devices. -
midi
– controls whether the current document is allowed to use the Web MIDI API. -
payment
– controls whether the current document is allowed to use the Payment Request API. -
vr
– controls whether the current document is allowed to use the WebVR API.
-
-
alternateText
- sets an alternate text for the frame in case the resource is not set or unavailable.
-
The
referrerpolicy
attribute indicates which referrer to send when fetching the frame’s resource.ReferrerPolicy
– enum of standard values of the attribute:-
no-referrer
– the referer header will not be sent. -
no-referrer-when-downgrade
– the referer header will not be sent to origins without TLS (HTTPS). -
origin
– the sent referrer will be limited to the origin of the referring page: its scheme, host, and port. -
origin-when-cross-origin
– the referrer sent to other origins will be limited to the scheme, the host, and the port. Navigation on the same origin will still include the path. -
same-origin
– a referrer will be sent for the same origin, but cross-origin requests will contain no referrer information. -
strict-origin
– only sends the origin of the document as the referrer when the protocol security level stays the same (HTTPS->HTTPS), but doesn’t send it to a less secure destination (HTTPS->HTTP). -
strict-origin-when-cross-origin
– sends a full URL when performing a same-origin request, only sends the origin when the protocol security level stays the same (HTTPS->HTTPS), and sends no header to a less secure destination (HTTPS->HTTP). -
unsafe-url
– the referrer will include the origin and the path. This value is unsafe because it leaks origins and paths from TLS-protected resources to insecure origins.
-
-
The
sandbox
attribute applies extra restrictions to the content in the frame. The value of the attribute should be either empty to apply all restrictions or space-separated tokens to lift particular restrictions.Sandbox
– enum of standard values of the attribute:-
allow-forms
– allows the resource to submit forms. -
allow-modals
– lets the resource open modal windows. -
allow-orientation-lock
– lets the resource lock the screen orientation. -
allow-pointer-lock
– lets the resource use the Pointer Lock API. -
allow-popups
– allows popups (such aswindow.open()
,target="_blank"
, orshowModalDialog()
). -
allow-popups-to-escape-sandbox
– lets the sandboxed document open new windows without those windows inheriting the sandboxing. -
allow-presentation
– lets the resource start a presentation session. -
allow-same-origin
– allows theiframe
content to be treated as being from the same origin. -
allow-scripts
– lets the resource run scripts. -
allow-storage-access-by-user-activation
– lets the resource request access to the parent’s storage capabilities with the Storage Access API. -
allow-top-navigation
– lets the resource navigate the top-level browsing context (the one named_top
). -
allow-top-navigation-by-user-activation
– lets the resource navigate the top-level browsing context, but only if initiated by a user gesture. -
allow-downloads-without-user-activation
– allows for downloads to occur without a gesture from the user. -
""
– applies all restrictions.
-
-
The
srcdoc
attribute – inline HTML to embed, overriding thesrc
attribute. The IE and Edge browsers don’t support this attribute. You can also specify a value for thesrcdoc
attribute using thesrcdocFile
attribute in xml by passing the path to the file with HTML code.
-
The
srcdocFile
attribute – the path to the file whose contents will be set to thesrcdoc
attribute. File content is obtained by using the classPath resource. You can set the attribute value only in the XML descriptor.
browserFrame
resources settings:
-
bufferSize
- the size of the download buffer in bytes used for this resource.<browserFrame> <file bufferSize="1024" path="C:/img.png"/> </browserFrame>
-
cacheTime
- the length of cache expiration time in milliseconds.<browserFrame> <file cacheTime="2400" path="C:/img.png"/> </browserFrame>
-
mimeType
- the MIME type of the resource.<browserFrame> <url url="https://avatars3.githubusercontent.com/u/17548514?v=4&s=200" mimeType="image/png"/> </browserFrame>
Methods of the BrowserFrame
interface:
-
addSourceChangeListener()
- adds a listener that will be notified when the content source is changed.@Inject private Notifications notifications; @Inject BrowserFrame browserFrame; @Subscribe protected void onInit(InitEvent event) { browserFrame.addSourceChangeListener(sourceChangeEvent -> notifications.create() .withCaption("Content updated") .show()); }
-
setSource()
- sets the content source for the frame. The method accepts the resource type and returns the resource object that can be configured using the fluent interface. Each resource type has its own methods, for example,setPath()
forThemeResource
type orsetStreamSupplier()
forStreamResource
type:BrowserFrame frame = uiComponents.create(BrowserFrame.NAME); try { frame.setSource(UrlResource.class).setUrl(new URL("http://www.foobar2000.org/")); } catch (MalformedURLException e) { throw new RuntimeException(e); }
You can use the same resource types as for the
Image
component.
-
createResource()
- creates the frame resource implementation by its type. The created object can be later passed to thesetSource()
method.UrlResource resource = browserFrame.createResource(UrlResource.class) .setUrl(new URL(fromString)); browserFrame.setSource(resource);
- HTML markup in BrowserFrame:
-
BrowserFrame
can be used to integrate HTML markup into your application. For example, you can dynamically generate HTML content at runtime from the user input.<textArea id="textArea" height="250px" width="400px"/> <browserFrame id="browserFrame" height="250px" width="500px"/>
textArea.addTextChangeListener(event -> { byte[] bytes = event.getText().getBytes(StandardCharsets.UTF_8); browserFrame.setSource(StreamResource.class) .setStreamSupplier(() -> new ByteArrayInputStream(bytes)) .setMimeType("text/html"); });
- PDF in BrowserFrame:
-
Besides HTML,
BrowserFrame
can be also used for displaying PDF files content. Use the file as a resource and set the corresponding MIME type for it:@Inject private BrowserFrame browserFrame; @Inject private Resources resources; @Subscribe protected void onInit(InitEvent event) { browserFramePdf.setSource(StreamResource.class) .setStreamSupplier(() -> resources.getResourceAsStream("/com/company/demo/" + "web/screens/CUBA_Hands_on_Lab_6.8.pdf")) .setMimeType("application/pdf"); }
- Attributes of browserFrame
-
align - allow - alternateText - caption - captionAsHtml - contextHelpText - contextHelpTextHtmlEnabled - colspan - css - description - descriptionAsHtml - enable - box.expandRatio - height - htmlSanitizerEnabled - icon - id - referrerpolicy - responsive - rowspan - sandbox - srcdoc - srcdocFile - stylename - visible - width
- Attributes of browserFrame resources
- Elements of browserFrame
-
classpath - file - relativePath - theme - url
- API