3.5.2.1.50. Tree
The Tree
component is intended to display hierarchical structures represented by entities referencing themselves.
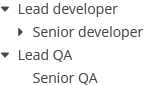
XML-name of the component: tree
Below is an example of the Tree
component description in a screen XML-descriptor:
<data readOnly="true">
<collection id="departmentsDc" class="com.company.sales.entity.Department" view="department-view">
<loader id="departmentsDl">
<query>
<![CDATA[select e from sales_Department e]]>
</query>
</loader>
</collection>
</data>
<layout>
<tree id="departmentsTree" dataContainer="departmentsDc" hierarchyProperty="parentDept"/>
</layout>
Here, the dataContainer attribute contains a reference to a collection container, and the hierarchyProperty
attribute defines the name of the entity attribute which is a reference to same entity.
The name of the entity attribute to be displayed in the tree can be set using the captionProperty
attribute. If this attribute is not defined, the screen will show the entity instance name.
The contentMode
attribute defines how the tree element captions should be displayed. There are three predefined content modes:
-
TEXT
- textual values are displayed as plain text. -
PREFORMATTED
- textual values are displayed as preformatted text. In this mode, newlines are preserved when rendered on the screen. -
HTML
- textual values are interpreted and displayed as HTML. Care should be taken when using this mode to avoid XSS issues.
The setItemCaptionProvider()
method sets a function for placing the name of the entity attribute as a caption for each item of the tree.
Selection in Tree
:
-
multiselect
attribute enables setting multiple selection mode for tree items. Ifmultiselect
istrue
, users can select multiple items using keyboard or mouse holding Ctrl or Shift keys. By default, multiple selection mode is switched off.
-
selectionMode
- sets the rows selection mode. There are 3 predefined selection modes:-
SINGLE
- single record selection. -
MULTI
- multiple selection as in any table. -
NONE
- selection is disabled.
Rows selection events can be tracked by
SelectionListener
. The origin of the selection event can be tracked using isUserOriginated() method.The
selectionMode
attribute has priority over the deprecatedmultiselect
attribute. -
The setItemClickAction()
method may be used to define an action that will be performed when a tree node is double-clicked.
Each tree item can have an icon on the left. Create an implementation of the Function
interface in the setIconProvider()
method in the screen controller:
@Inject
private Tree<Department> tree;
@Subscribe
protected void onInit(InitEvent event) {
tree.setIconProvider(department -> {
if (department.getParentDept() == null) {
return "icons/root.png";
}
return "icons/leaf.png";
});
}
You can react to expanding and collapsing tree items as follows:
@Subscribe("tree")
public void onTreeExpand(Tree.ExpandEvent<Task> event) {
notifications.create()
.withCaption("Expanded: " + event.getExpandedItem().getName())
.show();
}
@Subscribe("tree")
public void onTreeCollapse(Tree.CollapseEvent<Task> event) {
notifications.create()
.withCaption("Collapsed: " + event.getCollapsedItem().getName())
.show();
}
The Tree
component has a DescriptionProvider, that can render HTML if the contentMode
is set to ContentMode.HTML
. The result of the execution of this provider is sanitized if htmlSanitizerEnabled
attribute is set to true
.
The htmlSanitizerEnabled
attribute overrides the value of global cuba.web.htmlSanitizerEnabled configuration property.
For old screens, the Tree
component can be bound to a datasource instead of data container. In this case, the nested treechildren
element should be defined and contain a reference to a hierarchicalDatasource
in the datasource attribute. Declaration of a hierarchicalDatasource
should contain a hierarchyProperty
attribute – the name of the entity attribute which is a reference to same entity.
- Attributes of tree
-
caption - captionAsHtml - captionProperty - contentMode - contextHelpText - contextHelpTextHtmlEnabled - css - dataContainer - description - descriptionAsHtml - enable - box.expandRatio - height - htmlSanitizerEnabled - id - multiselect - showOrphans - stylename - tabIndex - visible - width
- Elements of tree
- Attributes of treechildren
- API
-
addCollapseListener - addExpandListener - setDescriptionProvider - setDetailsGenerator - setItemCaptionProvider