3.5.2.1.33. PopupView
PopupView
is a component that allows you to open a popup with a container. The popup can be opened by clicking on minimized value or programmatically. It can be closed by mouse out or by clicking on outside area.
A typical PopupView
with hidden and visible popup is shown below:
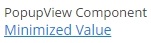
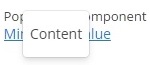
An example of a PopupView with minimized value retrieved from a localized message pack:
<popupView id="popupView"
minimizedValue="msg://minimizedValue"
caption="PopupView caption">
<vbox width="60px" height="40px">
<label value="Content" align="MIDDLE_CENTER"/>
</vbox>
</popupView>
The inner content of the PopupView
should be a container, for example BoxLayout.
PopupView
methods:
-
setPopupVisible()
allows you to open popup window programmatically.@Inject private PopupView popupView; @Subscribe protected void onInit(InitEvent event) { popupView.setMinimizedValue("Hello world!"); }
-
setMinimizedValue()
allows you to set a minimized value programmatically.@Inject private PopupView popupView; @Override public void init(Map<String, Object> params) { popupView.setMinimizedValue("Hello world!"); }
-
addPopupVisibilityListener(PopupVisibilityListener listener)
allows you to listen to the popup window visibility changes.@Inject private PopupView popupView; @Inject private Notifications notifications; @Subscribe protected void onInit(InitEvent event) { popupView.addPopupVisibilityListener(popupVisibilityEvent -> notifications.create() .withCaption(popupVisibilityEvent.isPopupVisible() ? "The popup is visible" : "The popup is hidden") .withType(Notifications.NotificationType.HUMANIZED) .show() ); }
-
The
PopupView
component has methods for setting the popup position. Thetop
andleft
values determine the position of the top-left corner of the popup. You can use standard values or set custom values of the position. The standard values are:-
TOP_RIGHT
-
TOP_LEFT
-
TOP_CENTER
-
MIDDLE_RIGHT
-
MIDDLE_LEFT
-
MIDDLE_CENTER
-
BOTTOM_RIGHT
-
BOTTOM_LEFT
-
BOTTOM_CENTER
The
DEFAULT
value sets the popup in the middle of the minimized value.These are the methods for setting the popup position:
-
void setPopupPosition(int top, int left)
- sets thetop
andleft
popup position. -
void setPopupPositionTop(int top)
- sets thetop
popup position. -
void setPopupPositionLeft(int left)
- sets theleft
popup position. -
void setPopupPosition(PopupPosition position)
- sets the popup position using standard values.@Inject private PopupView popupView; @Subscribe public void onInit(InitEvent event) { popupView.setPopupPosition(PopupView.PopupPosition.BOTTOM_CENTER); }
If the popup position is set using standard values, the
left
andtop
values will be reset and vice versa.
-
-
The popup set with one of the standard values will be displayed with a slight indentation. You can disable this indent by overriding
$popup-horizontal-margin
and$popup-vertical-margin
variables in styles. -
To get the popup position use the following methods:
-
int getPopupPositionTop()
- returns thetop
popup position. -
int getPopupPositionLeft()
- returns theleft
popup position. -
PopupPosition getPopupPosition()
- returns null in case the popup position is set without using standard values.
-
PopupView
attributes:
-
minimizedValue
attribute defines the text of popup button. This text may contain HTML tags.
-
If the
hideOnMouseOut
attribute is set tofalse
, popup container will close after click on outside area.
- Attributes of popupView
-
caption - captionAsHtml - contextHelpText - contextHelpTextHtmlEnabled - css - description - descriptionAsHtml - box.expandRatio - height - htmlSanitizerEnabled - hideOnMouseOut - icon - id - minimizedValue - stylename - visible - width
- API