5.5.2.1.12. FileMultiUploadField
The FileMultiUploadField
component allows a user to upload files to the server. The component is a button; when it is clicked, a standard OS file picker window is shown, where the user can select multiple files for upload.
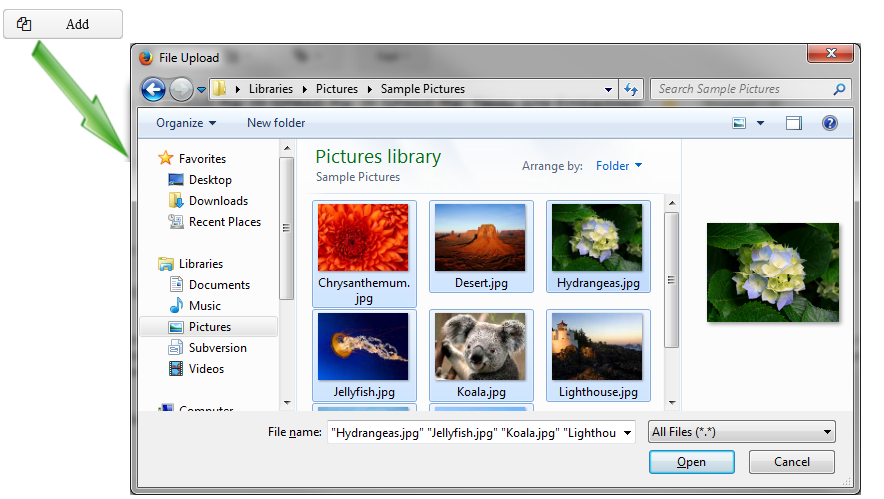
XML name of the component: multiUpload
.
The component is implemented for Web Client and Desktop Client.
-
Declare the component in an XML screen descriptor:
<multiUpload id="multiUploadField" caption="msg://upload"/>
-
In the screen controller, inject the component itself, the FileUploadingAPI and DataSupplier interfaces. In the
init()
method, add listeners which will react on successful uploads and errors:@Inject private FileMultiUploadField multiUploadField; @Inject private FileUploadingAPI fileUploadingAPI; @Inject private DataSupplier dataSupplier; @Override public void init(Map<String, Object> params) { multiUploadField.addQueueUploadCompleteListener(() -> { for (Map.Entry<UUID, String> entry : multiUploadField.getUploadsMap().entrySet()) { UUID fileId = entry.getKey(); String fileName = entry.getValue(); FileDescriptor fd = fileUploadingAPI.getFileDescriptor(fileId, fileName); // save file to FileStorage try { fileUploadingAPI.putFileIntoStorage(fileId, fd); } catch (FileStorageException e) { new RuntimeException("Error saving file to FileStorage", e); } // save file descriptor to database dataSupplier.commit(fd); } showNotification("Uploaded files: " + multiUploadField.getUploadsMap().values(), NotificationType.HUMANIZED); multiUploadField.clearUploads(); }); multiUploadField.addFileUploadErrorListener(event -> showNotification("File upload error", NotificationType.HUMANIZED)); }
The component uploads all selected files to the temporary storage of the client tier and invokes the listener added by the
addQueueUploadCompleteListener()
method. In this listener, theFileMultiUploadField.getUploadsMap()
method is invoked to obtain a map of temporary storage file identifiers to file names. Then, correspondingFileDescriptor
objects are created by callingFileUploadingAPI.getFileDescriptor()
for each map entry.com.haulmont.cuba.core.entity.FileDescriptor
(do not confuse withjava.io.FileDescriptor
) is a persistent entity, which uniquely identifies an uploaded file and then is used to download the file from the system.Below is the list of listeners available to track the upload process:
-
FileUploadStartListener
,
-
FileUploadStartListener
,
-
FileUploadFinishListener
, -
QueueUploadCompleteListener
.
FileUploadingAPI.putFileIntoStorage()
method is used to move the uploaded file from the temporary client storage to FileStorage. Parameters of this method are temporary storage file identifier and theFileDescriptor
object.After uploading the file to
FileStorage
, theFileDescriptor
instance is saved in a database by invokingDataSupplier.commit()
. The saved instance returned by this method can be set to an attribute of an entity related to this file. Here,FileDescriptor
is simply stored in the database. The file will be available through the Administration → External Files screen.After processing, the list of files should be cleared by calling the
clearUploads()
method in order to prepare for further uploads. -
-
Maximum upload size is determined by the cuba.maxUploadSizeMb application property and is 20MB by default. If a user selects a file of a larger size, a corresponding message will be displayed, and the upload will be interrupted.
-
The
accept
XML attribute (and the correspondingsetAccept()
method) can be used to set the file type mask in the file selection dialog. Users still be able to change the mask to "All files" and upload arbitrary files.The value of the attribute should be a comma-separated list of masks. For example:
*.jpg,*.png
.
-
The
fileSizeLimit
XML attribute (and the correspondingsetFileSizeLimit()
method) can be used to set maximum allowed file size in bytes. This limit defines a maximum size for each file.<multiUpload id="multiUploadField" fileSizeLimit="200000"/>
-
The
permittedExtensions
XML attribute (and the correspondingsetPermittedExtensions()
method) sets the white list of permitted file extensions.The value of the attribute should be a set of string values, where each string is a permitted extension with leading dot. For example:
uploadField.setPermittedExtensions(Sets.newHashSet(".png", ".jpg"));
-
The
dropZone
XML attribute allows you to specify a BoxLayout to be used as a target for drag-and-dropping files from outside of the browser. If the container style is not set, the selected container is highlighted when a user drags files over the container, otherwise the dropZone is not visible.
See Loading and Displaying Images for more complex example of working with uploaded files.
- Attributes of multiUpload
-
accept - align - caption - description - dropZone - enable - fileSizeLimit - height - icon - id - permittedExtensions - stylename - tabIndex - visible - width
- Listeners of multiUpload
-
FileUploadErrorListener - FileUploadFinishListener - FileUploadStartListener - QueueUploadCompleteListener