5.5.9. Desktop Client Specifics
Implementation of the generic user interface in the Desktop Client block is based on Java Swing. The main classes available in the desktop client infrastructure are described below.
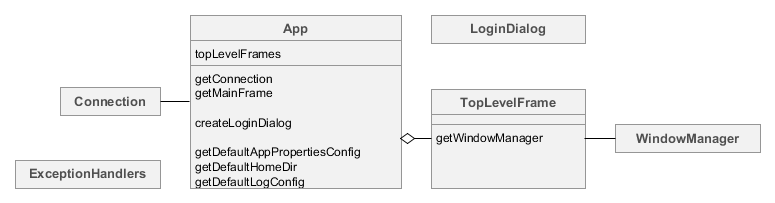
-
App
– central class of the desktop application infrastructure. Contains links toConnection
and mainTopLevelFrame
, as well as methods for initialization and retrieval of application settings.In your application, you should create a custom class – inheritor of
App
and override the following methods:-
getDefaultAppPropertiesConfig()
- should return a string where all application properties files should be listed separated by spaces:@Override protected String getDefaultAppPropertiesConfig() { return "/cuba-desktop-app.properties /desktop-app.properties"; }
-
getDefaultHomeDir()
- should return path to the folder, where temporary and work files should be stored. For example:@Override protected String getDefaultHomeDir() { return System.getProperty("user.home") + "/.mycompany/sales"; }
-
getDefaultLogConfig()
- should return name of the Logback configuration file, if it is defined for the project. For example:@Override protected String getDefaultLogConfig() { return "sales-logback.xml"; }
Additionally, for your custom class inheriting from the
App
you should definemain()
method in the following way:public static void main(final String[] args) { SwingUtilities.invokeLater(new Runnable() { public void run() { app = new App(); app.init(args); app.show(); app.showLoginDialog(); } }); }
-
-
Connection
- is a class that provides the functionality of connecting to middleware and storing a user session. -
LoginDialog
– the dialog to enter credentials. In your application you can create an inheritor ofLoginDialog
and redefine thecreateLoginDialog()
method of theApp
class to use it. -
TopLevelFrame
– inheritor ofJFrame
, which is the top level window. The application has at least one instance of this class created when application is started and containing the main menu. This instance is returned by thegetMainFrame()
method of theApp
class.When a user detaches tabs from the main window or a TabSheet (see
detachable
attribute), additional instances ofTopLevelFrame
that do not contain main menu are created. -
WindowManager
- the central class implementing application screens management logic.openEditor()
,showMessageDialog()
and other methods of theFrame
interface implemented by screen controllers delegate to the window manager.WindowManager
class is located in the platform’s common gui module and is abstract. The desktop desktop module has a dedicatedDesktopWindowManager
class that implements desktop client specifics.Typically,
WindowManager
is not used in the application code directly. -
ExceptionHandlers
- contains a collection of client-level exception handlers.