4.5.2.1.3. Calendar
The Calendar
component is intended to organize and display calendar events.
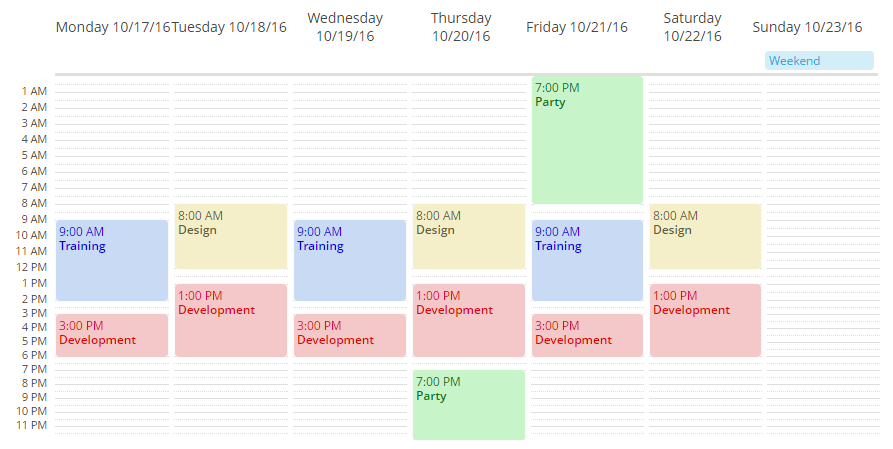
XML-name of the component: calendar
.
The component is implemented for Web Client.
An example of component definition in an XML-descriptor of a screen:
<calendar id="calendar"
captionProperty="caption"
startDate="2016-10-01"
endDate="2016-10-31"
height="100%"
width="100%"/>
The view mode is determined from the date range of the calendar, defined by the start date and the end date. The default view is the weekly view, it is used for ranges up to seven days a week. For a single-day view use the range within one date. Calendar will be shown in a monthly view when the date range is over than one week (seven days) long.
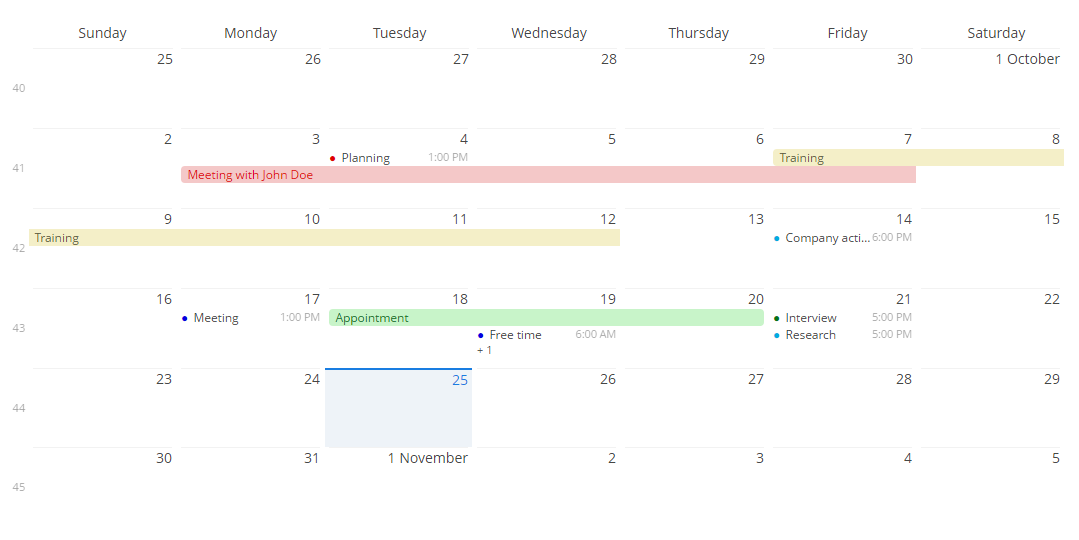
Attributes of calendar
:
-
endDate
- the end date for the calendar’s range.
-
endDateProperty
- the name of an entity attribute that contains the end date.
-
descriptionProperty
- the name of an entity attribute that contains the event description.
-
isAllDayProperty
- the name of an entity attribute that determines if the event is all day long.
-
startDate
- the start date for the calendar’s range.
-
startDateProperty
- the name of an entity attribute that contains the start date.
-
stylenameProperty
- the name of an entity attribute that contains the event style name.
-
timeFormat
- time format: 12H or 24H.
To display events in the calendar cells, you can add the events directly to the Calendar
object using the addEvent()
method or use the CalendarEventProvider
interface. An example of direct event adding:
@Inject
private Calendar calendar;
public void generateEvent(String caption, String description, Date start, Date end, boolean isAllDay, String stylename) {
SimpleCalendarEvent calendarEvent = new SimpleCalendarEvent();
calendarEvent.setCaption(caption);
calendarEvent.setDescription(description);
calendarEvent.setStart(start);
calendarEvent.setEnd(end);
calendarEvent.setAllDay(isAllDay);
calendarEvent.setStyleName(stylename);
calendar.getEventProvider().addEvent(calendarEvent);
}
There are two data providers available: ListCalendarEventProvider
(created by default) and EntityCalendarEventProvider
.
ListCalendarEventProvider
is filled by addEvent()
method that gets a CalendarEvent
object as a parameter:
@Inject
private Calendar calendar;
public void addEvents() {
ListCalendarEventProvider listCalendarEventProvider = new ListCalendarEventProvider();
calendar.setEventProvider(listCalendarEventProvider);
listCalendarEventProvider.addEvent(generateEvent("Training", "Student training", "2016-10-17 09:00", "2016-10-17 14:00", false, "event-blue"));
listCalendarEventProvider.addEvent(generateEvent("Development", "Platform development", "2016-10-17 15:00", "2016-10-17 18:00", false, "event-red"));
listCalendarEventProvider.addEvent(generateEvent("Party", "Party with friends", "2016-10-22 13:00", "2016-10-22 18:00", false, "event-yellow"));
}
private SimpleCalendarEvent generateEvent(String caption, String description, String start, String end, Boolean allDay, String style) {
SimpleCalendarEvent calendarEvent = new SimpleCalendarEvent();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm");
calendarEvent.setCaption(caption);
calendarEvent.setDescription(description);
calendarEvent.setStart(df.parse(start));
calendarEvent.setEnd(df.parse(end));
calendarEvent.setAllDay(allDay);
calendarEvent.setStyleName(style);
return calendarEvent;
}
EntityCalendarEventProvider
is filled with data directly from an entity fields. To be used for the EntityCalendarEventProvider
, an entity should at least have attributes for the event start date (DateTime type), event end date (DateTime type) and event caption (String type). In the example below we will assume that the datasource entity has all required attributes: eventCaption
, eventDescription
, eventStartDate
, eventEndDate
, eventStylename
, and will set their names as values for calendar
attributes:
<calendar id="calendar"
datasource="calendarEventsDs"
width="100%"
height="100%"
startDate="2016-10-01"
endDate="2016-10-31"
captionProperty="eventCaption"
descriptionProperty="eventDescription"
startDateProperty="eventStartDate"
endDateProperty="eventEndDate"
stylenameProperty="eventStylename"/>
The Calendar
component supports several event listeners for user interaction with its elements, such as date and week captions, date/time range selections, event dragging and event resizing. Navigation buttons used to scroll forward and backward in time are also listened by the server. Below is the list of default listeners:
-
addDateClickListener(CalendarDateClickListener listener);
- adds listener for date clicks:calendar.addDateClickListener( calendarDateClickEvent -> showNotification(String.format("Date clicked: %s", calendarDateClickEvent.getDate().toString()), NotificationType.HUMANIZED ) );
-
addWeekClickListener()
- adds listener for week number clicks. -
addEventClickListener()
- adds listener for calendar event clicks. -
addEventResizeListener()
- adds listener for event size changing. -
addEventMoveListener()
- adds listener for event drag and drop. -
addForwardClickListener()
- adds listener for calendar forward scrolling. -
addBackwardClickListener()
- adds listener for calendar backward scrolling.
Calendar events can be styled with CSS. To configure a style, create the style name and set the parameters in the .scss
-file. For example, let’s configure the background color of an event:
.v-calendar-event.event-green {
background-color: #c8f4c9;
color: #00e026;
}
Then use the setStyleName
method of the event:
calendarEvent.setStyleName("event-green");
As a result, the event’s background is green:
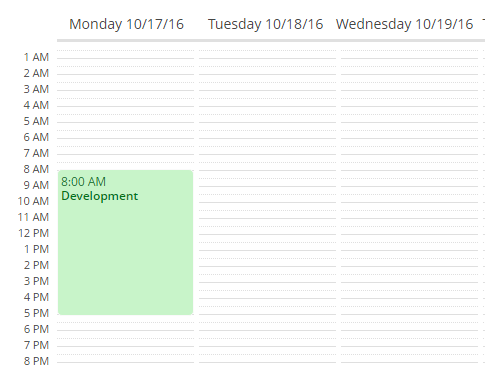
- Attributes of calendar
-
caption - captionProperty - colspan - datasource - description - descriptionProperty - endDateProperty - endDate - height - icon - id - isAllDayProperty - rowspan - startDate - startDateProperty - stylename - stylenameProperty - timeFormat - visible - width