5.5.2.1.25. OptionsGroup
This is a component that allows a user to choose from a list of options. Radio buttons are used to select a single value; a group of checkboxes is used to select multiple values.
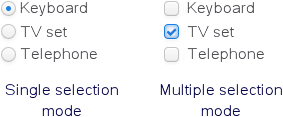
XML name of the component: optionsGroup
.
The OptionsGroup
component is implemented for Web Client and Desktop Client.
-
The simplest case of using
OptionsGroup
is to select an enumeration value for an entity attribute. For example, aRole
entity hastype
attribute of theRoleType
type, which is an enumeration. Then you can useOptionsGroup
to edit this attribute as follows:<dsContext> <datasource id="roleDs" class="com.haulmont.cuba.security.entity.Role" view="_local"/> </dsContext> <layout> <optionsGroup datasource="roleDs" property="type"/>
In the example above
roleDs
data source is defined for theRole
entity. In theoptionsGroup
component, the link to the data source is specified in the datasource attribute and the name of the entity attribute is set in the property attribute.As a result, the component will be as follows:
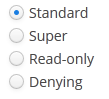
-
The list of component options can be specified arbitrarily using the
setOptionsList()
,setOptionsMap()
andsetOptionsEnum()
methods, or using anoptionsDatasource
attribute.
-
setOptionsList()
allows you to specify programmatically a list of component options. To do this, declare a component in the XML descriptor:<optionsGroup id="numberOfSeatsField"/>
Then inject the component into the controller and specify a list of options in the
init()
method:@Inject protected OptionsGroup numberOfSeatsField; @Override public void init(Map<String, Object> params) { List<Integer> list = new ArrayList<>(); list.add(2); list.add(4); list.add(5); list.add(7); numberOfSeatsField.setOptionsList(list); }
The component will be as follows:
Depending on the selected option, the
getValue()
method of the component will returnInteger
values: 2, 4, 5, 7.
-
setOptionsMap()
allows you to specify string names and option values separately. For example, we can set the following options map for thenumberOfSeatsField
component, described the XML descriptor, in theinit()
method of the controller:@Inject protected OptionsGroup numberOfSeatsField; @Override public void init(Map<String, Object> params) { Map<String, Object> map = new LinkedHashMap<>(); map.put("two", 2); map.put("four", 4); map.put("five", 5); map.put("seven", 7); numberOfSeatsField.setOptionsMap(map); }
The component will be as follows:
Depending on the selected option, the
getValue()
method of the component will returnInteger
values: 2, 4, 5, 7, and not the strings that are displayed on the screen.
-
setOptionsEnum()
takes a class of enumeration as a parameter. The options list will consist of localized names of enum values, the value of the component will be an enum value. -
The component can take a list of options from a data source. For this purpose, the optionsDatasource attribute is used. For example:
<dsContext> <collectionDatasource id="coloursDs" class="com.company.sample.entity.Colour" view="_local"> <query>select c from sample$Colour c</query> </collectionDatasource> </dsContext> <layout> <optionsGroup id="coloursField" optionsDatasource="coloursDs"/>
In this case, the
coloursField
component will display instance names of theColour
entity, located in thecoloursDs
data source, and itsgetValue()
method will return the selected entity instance.With the help of captionProperty attribute entity attribute to be used instead of an instance name for string option names can be defined.
-
multiselect
attribute is used to switchOptionsGroup
to a multiple choice mode. Ifmultiselect
is turned on, the component is displayed as a group of independent checkboxes, and the component value is a list of selected options.For example, if we create the component in the XML screen descriptor:
<optionsGroup id="roleTypesField" multiselect="true"/>
and set a list of options for it –
RoleType
enumeration values:@Inject protected OptionsGroup roleTypesField; @Override public void init(Map<String, Object> params) { roleTypesField.setOptionsList(Arrays.asList(RoleType.values())); }
then the component will be as follows:
In this case the
getValue()
method of the component will return ajava.util.List
, containingRoleType.READONLY
andRoleType.DENYING
values.The example above also illustrates an ability of the
OptionsGroup
component to display localized values of enumerations included in the data model.
-
The
orientation
attribute defines the orientation of group elements. By default, elements are arranged vertically. Thehorizontal
value sets the horizontal orientation.
- Attributes of optionsGroup
-
align - caption - captionProperty - datasource - description - editable - enable - icon - id - multiselect - height - optionsDatasource - orientation - property - required - requiredMessage - stylename - tabIndex - visible - width
- Elements of optionsGroup
- API
-
addValueChangeListener - setOptionsEnum - setOptionsList - setOptionsMap