5.5.2.1.38. SuggestionPickerField
The SuggestionPickerField
component is designed to search for entity instances according to a string entered by a user. It differs from SearchPickerField in that it refreshes the list of options on each entered symbol without the need to press Enter. The list of options is loaded in background according to the logic defined by the application developer on the server side.
SuggestionPickerField
is also a PickerField and can contain actions represented by buttons on the right.
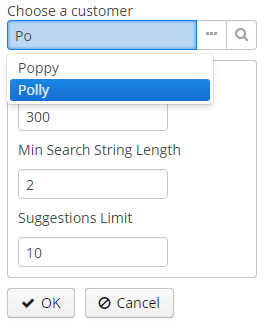
XML name of the component: suggestionPickerField
.
The component is implemented for the Web Client.
SuggestionPickerField
is used to select reference entity attributes, so you usually set its datasource
and property
attributes:
<dsContext>
<datasource id="orderDs"
class="com.company.sample.entity.Order"
view="order-view"/>
</dsContext>
<layout>
<suggestionPickerField id="suggestionPickerField"
captionProperty="name"
datasource="orderDs"
property="customer"/>
</layout>
suggestionPickerField
attributes:
-
asyncSearchDelayMs
- sets the delay between the last key press action and asynchronous search.
-
metaClass
- sets the link to the component’sMetaClass
if the component is used without binding to a datasource, i.e., without setting datasource and property.
-
minSearchStringLength
- sets the minimal string length which is required to perform suggestions search.
-
popupWidth
- sets the width of the suggestion popup.Possible options:
-
auto
- the popup width will be equal to the maximum width of suggestions, -
parent
- the popup width will be equal to the width of main component, -
absolute (e.g.
"170px"
) or relative (e.g."50%"
) value.
-
-
suggestionsLimit
- sets the limit of suggestions to be displayed.
Tip
|
The appearance of |
suggestionPickerField
elements:
-
actions
- an optional element describing the actions related to the component. In addition to custom arbitrary actions,suggestionPickerField
supports the following standard actions, defined in thePickerField.ActionType
enumeration:lookup
,open
,clear
.
- Base SuggestionPickerField usage
-
In the most common case, it is sufficient to set
SearchExecutor
to the component.SearchExecutor
is a functional interface that contains a single method:List<E extends Entity> search(String searchString, Map<String, Object> searchParams)
:suggestionPickerField.setSearchExecutor((searchString, searchParams) -> { return Arrays.asList(entity1, entity2, ...); });
WarningThe
search()
method is executed in a background thread so it cannot access visual components or datasources used by visual components. Call DataManager or a middleware service directly; or process and return data loaded to the screen beforehand.The
searchString
parameter can be used to filter candidates using the string entered by the user. You can use theescapeForLike()
method to search for the values that contain special symbols:suggestionPickerField.setSearchExecutor((searchString, searchParams) -> { searchString = QueryUtils.escapeForLike(searchString); return dataManager.loadList(LoadContext.create(Customer.class).setQuery( LoadContext.createQuery("select c from sample$Customer c where c.name like :name order by c.name escape '\\'") .setParameter("name", "%" + searchString + "%"))); });
- ParametrizedSearchExecutor usage
-
In the previous examples,
searchParams
is an empty map. To define params, you should useParametrizedSearchExecutor
:suggestionPickerField.setSearchExecutor(new SuggestionField.ParametrizedSearchExecutor<Customer>(){ @Override public Map<String, Object> getParams() { return ParamsMap.of(...); } @Override public List<Customer> search(String searchString, Map<String, Object> searchParams) { return executeSearch(searchString, searchParams); } });
- EnterActionHandler and ArrowDownActionHandler usage
-
Another way to use the component is to set
EnterActionHandler
orArrowDownActionHandler
. These listeners are fired when a user presses Enter or Arrow Down keys when the popup with suggestions is hidden. They are also functional interfaces with single method and single parameter -currentSearchString
. You can set up your own action handlers and useSuggestionField.showSuggestions()
method which accepts the list of entities to show suggestions.suggestionPickerField.setArrowDownActionHandler(currentSearchString -> { List<Customer> suggestions = findSuggestions(); suggestionPickerField.showSuggestions(suggestions); }); suggestionPickerField.setEnterActionHandler(currentSearchString -> { List<Customer> suggestions = getDefaultSuggestions(); suggestionPickerField.showSuggestions(suggestions); });
- Attributes of suggestionPickerField
-
align - asyncSearchDelayMs - caption - captionProperty - colspan - contextHelpText - contextHelpTextHtmlEnabled - datasource - description - editable - enable - height - icon - id - inputPrompt - metaClass - minSearchStringLength - popupWidth - property - required - requiredMessage - responsive - rowspan - stylename - suggestionsLimit - tabIndex - visible - width
- Elements of suggestionPickerField
- Predefined styles of suggestionPickerField
- API
-
addValueChangeListener - setContextHelpIconClickHandler - setOptionsStyleProvider