5.5.2.1.2. BrowserFrame
A BrowserFrame
is designed to display embedded web pages. It is the equivalent of the HTML iframe
element.
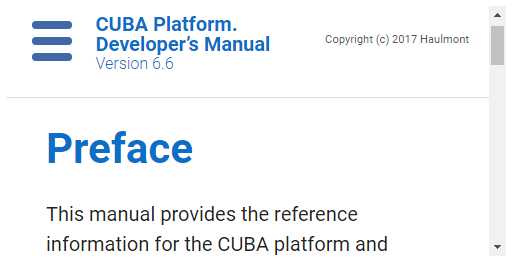
Component’s XML-name: browserFrame
The component is implemented for Web Client.
An example of component definition in an XML-descriptor of a screen:
<browserFrame id="browserFrame"
height="250px"
width="500px"
align="MIDDLE_CENTER">
<url url="https://doc.cuba-platform.com/manual-6.6/"/>
</browserFrame>
Similarly to the Image component, the BrowserFrame
component can also display images from different resources. You can set the resource type declaratively using the browserFrame
elements listed below:
-
classpath
- a resource in the classpath.<browserFrame> <classpath path="com/company/sample/web/screens/myPic.jpg"/> </browserFrame>
-
file
- a resource in the file system.<browserFrame> <file path="D:\sample\modules\web\web\VAADIN\images\myImage.jpg"/> </browserFrame>
-
relativePath
- resource in the application directory.<browserFrame> <relativePath path="VAADIN/images/myImage.jpg"/> </browserFrame>
-
theme
- a theme resource, for example:<browserFrame> <theme path="../halo/com.company.demo/myPic.jpg"/> </browserFrame>
-
url
- a resource which can be loaded from the given URL.<browserFrame> <url url="http://www.foobar2000.org/"/> </browserFrame>
browserFrame
attributes:
-
alternateText
- sets an alternate text for the frame in case the resource is not set or unavailable.
browserFrame
resources settings:
-
bufferSize
- the size of the download buffer in bytes used for this resource.<browserFrame> <file bufferSize="1024" path="C:/img.png"/> </browserFrame>
-
cacheTime
- the length of cache expiration time in milliseconds.<browserFrame> <file cacheTime="2400" path="C:/img.png"/> </browserFrame>
-
mimeType
- the MIME type of the resource.<browserFrame> <url url="https://avatars3.githubusercontent.com/u/17548514?v=4&s=200" mimeType="image/png"/> </browserFrame>
Methods of the BrowserFrame
interface:
-
addSourceChangeListener()
- adds a listener that will be notified when the content source is changed.browserFrame.addSourceChangeListener(event -> showNotification("Content updated"));
-
setSource()
- sets the content source for the frame. The method accepts the resource type and returns the resource object that can be configured using the fluent interface. Each resource type has its own methods, for example,setPath()
forThemeResource
type orsetStreamSupplier()
forStreamResource
type:BrowserFrame frame = componentsFactory.createComponent(BrowserFrame.class); try { frame.setSource(UrlResource.class).setUrl(new URL("http://www.foobar2000.org/")); } catch (MalformedURLException e) { throw new RuntimeException(e); }
You can use the same resource types as for the
Image
component.
-
createResource()
- creates the frame resource implementation by its type. The created object can be later passed to thesetSource()
method.UrlResource resource = browserFrame.createResource(UrlResource.class) .setUrl(new URL(fromString)); browserFrame.setSource(resource);
- HTML markup in BrowserFrame:
-
BrowserFrame
can be used to integrate HTML markup into your application. For example, you can dynamically generate HTML content at runtime from the user input.<textArea id="textArea" height="250px" width="400px"/> <browserFrame id="browserFrame" height="250px" width="500px"/>
textArea.addTextChangeListener(event -> { byte[] bytes = event.getText().getBytes(StandardCharsets.UTF_8); browserFrame.setSource(StreamResource.class) .setStreamSupplier(() -> new ByteArrayInputStream(bytes)) .setMimeType("text/html"); });
- PDF in BrowserFrame:
-
Besides HTML,
BrowserFrame
can be also used for displaying PDF files content. Use the file as a resource and set the corresponding MIME type for it:@Inject private BrowserFrame browserFrame; @Inject private Resources resources; @Override public void init(Map<String, Object> params) { browserFramePdf.setSource(StreamResource.class) .setStreamSupplier(() -> resources.getResourceAsStream("/com/company/demo/" + "web/screens/CUBA_Hands_on_Lab_6.8.pdf")) .setMimeType("application/pdf"); }
- Attributes of browserFrame
-
align - alternateText - caption - colspan - description - enable - height - icon - id - responsive - rowspan - stylename - visible - width
- Attributes of browserFrame resources
- Elements of browserFrame
-
classpath - file - relativePath - theme - url
- API