5.5.2.1.23. LookupField
This is a component to select a value from drop-down list. Drop-down list provides the filtering of values as the user inputs some text, and the pagination of available values.
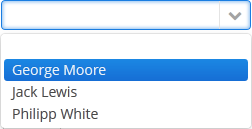
XML name of the component: lookupField
.
The LookupField
component is implemented for Web Client and Desktop Client.
-
The simplest case of using
LookupField
is to select an enumeration value for an entity attribute. For example, aRole
entity has atype
attribute of theRoleType
type, which is an enumeration. Then you can useLookupField
to edit this attribute as follows:<dsContext> <datasource id="roleDs" class="com.haulmont.cuba.security.entity.Role" view="_local"/> </dsContext> <layout> <lookupField datasource="roleDs" property="type"/>
In the example above, the screen defines
roleDs
data source for theRole
entity. In thelookupField
component, a link to a data source is specified in the datasource attribute, and a name of an entity attribute is specified in the property attribute. In this case, the entity attribute is an enumeration and the drop-down list will display localized names of all enumeration values. -
Similarly,
LookupField
can be used to select an instance of a related entity. optionsDatasource attribute is used to create a list of options:<dsContext> <datasource id="carDs" class="com.company.sample.entity.Car" view="_local"/> <collectionDatasource id="coloursDs" class="com.company.sample.entity.Colour" view="_local"> <query>select c from sample$Colour c</query> </collectionDatasource> </dsContext> <layout> <lookupField datasource="carDs" property="colour" optionsDatasource="coloursDs"/>
In this case, the component will display instance names of the
Colour
entity located in thecolorsDs
data source, and the selected value will be set into thecolour
attribute of theCar
entity, which is located in thecarDs
data source.captionProperty attribute defines which entity attribute can be used instead of an instance name for string option names.
-
The list of component options can be specified arbitrarily using the
setOptionsList()
,setOptionsMap()
andsetOptionsEnum()
methods, or using the XMLoptionsDatasource
attribute.-
setOptionsList()
allows you to programmatically specify a list of component options. To do this, declare a component in the XML descriptor:<lookupField id="numberOfSeatsField" datasource="modelDs" property="numberOfSeats"/>
Then inject the component into the controller and specify a list of options in the
init()
method:@Inject protected LookupField numberOfSeatsField; @Override public void init(Map<String, Object> params) { List<Integer> list = new ArrayList<>(); list.add(2); list.add(4); list.add(5); list.add(7); numberOfSeatsField.setOptionsList(list); }
In the component’s drop-down list the values 2, 4, 5 and 7 will be displayed. Selected number will be put into the
numberOfSeats
attribute of an entity located in themodelDs
data source.
-
setOptionsMap()
allows you to specify string names and option values separately. For example, in thenumberOfSeatsField
component in the XML descriptor, specify an option map ininit()
:@Inject protected LookupField numberOfSeatsField; @Override public void init(Map<String, Object> params) { Map<String, Object> map = new LinkedHashMap<>(); map.put("two", 2); map.put("four", 4); map.put("five", 5); map.put("seven", 7); numberOfSeatsField.setOptionsMap(map); }
In the component’s drop-down list,
two
,four
,five
,seven
strings will be displayed. However, the value of the component will be a number that corresponds to the selected option. It will be put into thenumberOfSeats
attribute of an entity located in themodelDs
data source.
-
setOptionsEnum()
takes the class of an enumeration as a parameter. The drop-down list will show localized names of enum values, the value of the component will be an enum value.
-
-
OptionsStyleProvider
enables you to use separate style names for the the options displayed by the component using thesetOptionsStyleProvider()
method:lookupField.setOptionsStyleProvider((field, item) -> { User user = (User) item; switch (user.getGroup().getName()) { case "Company": return "company"; case "Premium": return "premium"; default: return "company"; } });
-
Each drop-down list component can have an icon on the left. Create an implementation of the
LookupField.OptionIconProvider
interface in the screen controller and set it for thelookupField
:lookupField.setOptionIconProvider(new LookupField.OptionIconProvider<Customer>(){ @Override public String getItemIcon(Customer c){ if(c.getType()== LegalStatus.LEGAL) return"icons/icon-office.png"; return"icons/icon-user.png"; } });
TipIf you use SVG icons, set the icon size explicitly to avoid icons overlay.
<svg version="1.1" id="Capa_1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" xml:space="preserve" style="enable-background:new 0 0 55 55;" viewBox="0 0 55 55" height="25px" width="25px">
-
If the
LookupField
component is not required and if the related entity attribute is not declared as required, the list of component options has an empty row. If this row is selected, the component returnsnull
. The nullName attribute allows you to specify a row to be displayed in this case instead of an empty one. Below is an example:<lookupField datasource="carDs" property="colour" optionsDatasource="coloursDs" nullName="(none)"/>
In this case, instead of an empty row,
(none)
will be displayed. If this row is selected,null
will be set to a related entity attribute.If you specify a list of options programmatically using
setOptionsList()
, you can pass one of the options intosetNullOption()
method. Then, if the user selects it, the component value will benull
.- LookupField filters:
-
-
Using the
filterMode
attribute, option filtering type can be defined for the user input:-
NO
− no filtering. -
STARTS_WITH
− by the beginning of a phrase. -
CONTAINS
− by any occurrence (is used by default).
-
-
The
setFilterPredicate()
method enables to setup how items should be filtered. The predicate tests whether an item with the given caption matches to the given search string. For example:BiFunction<String, String, Boolean> predicate = String::contains; lookupField.setFilterPredicate((itemCaption, searchString) -> predicate.apply(itemCaption.toLowerCase(), searchString));
The functional interface
FilterPredicate
has thetest
method that enables implementing custom filtering logic, for example, to escape accents or special characters:lookupField.setFilterPredicate((itemCaption, searchString) -> StringUtils.replaceChars(itemCaption, "ÉÈËÏÎ", "EEEII") .toLowerCase() .contains(searchString));
-
-
The
LookupField
component is able to handle user input if there is no suitable option in the list. In this case,setNewOptionAllowed()
andsetNewOptionHandler()
are used. For example:@Inject private Metadata metadata; @Inject protected LookupField colourField; @Inject protected CollectionDatasource<Colour, UUID> coloursDs; @Override public void init(Map<String, Object> params) { colourField.setNewOptionAllowed(true); colourField.setNewOptionHandler(new LookupField.NewOptionHandler() { @Override public void addNewOption(String caption) { Colour colour = metadata.create(Colour.class); colour.setName(caption); coloursDs.addItem(colour); colourField.setValue(colour); } }); }
The
NewOptionHandler
handler is invoked if the user enters a value that does not coincide with any option and presses Enter. In this case, a newColour
entity instance is created in the handler, itsname
attribute is set to the value entered by the user, this instance is added to the options data source and selected in the component.Instead of implementing the
LookupField.NewOptionHandler
interface for processing user input, the controller method name can be specified in thenewOptionHandler
XML-attribute. This method should have two parameters, one ofLookupField
type, and the other ofString
type. They will be set to the component instance and the value entered by the user, accordingly. ThenewOptionAllowed
attribute is used to enable adding new options instead of thesetNewOptionAllowed()
method.
-
The
nullOptionVisible
XML attribute sets visibility for the null option in the drop-down list. It allows you to makeLookupField
not required but still without the null option.
-
The
textInputAllowed
XML attribute can be used to disable filtering options from keyboard. It can be convenient for short lists. The default value istrue
.
-
The
pageLength
XML attribute allows you to redefine the number of options on one page of the drop-down list, specified in the cuba.gui.lookupFieldPageLength application property. -
In Web Client with a Halo-based theme, you can set predefined styles to the
LookupField
component using thestylename
attribute either in the XML descriptor or in the screen controller:<lookupField id="lookupField" stylename="borderless"/>
When setting a style programmatically, select one of the
HaloTheme
class constants with theLOOKUPFIELD_
prefix:lookupField.setStyleName(HaloTheme.LOOKUPFIELD_BORDERLESS);
LookupField styles:
-
align-center
- align the text inside the field to center.
-
align-right
- align the text inside the field to the right.
-
borderless
- removes the border and background from the text field.
-
- Attributes of lookupField
-
align - caption - captionProperty - contextHelpText - contextHelpTextHtmlEnabled - datasource - description - editable - enable - filterMode - height - icon - id - inputPrompt - newOptionAllowed - newOptionHandler - nullName - nullOptionVisible - optionsDatasource - optionsEnum - pageLength - property - required - requiredMessage - stylename - tabIndex - textInputAllowed - visible - width
- Elements of lookupField
- Predefined styles of lookupField
-
align-right - align-center - borderless - huge - large - small - tiny
- API
-
addValueChangeListener - commit - discard - isModified - setContextHelpIconClickHandler - setFilterPredicate - setOptionsEnum - setOptionsList - setOptionsMap - setOptionsStyleProvider