4.5.2.1.19. PickerField
PickerField
displays an entity instance in a text field and performs actions when a user clicks buttons on the right.

XML name of the component: pickerField
.
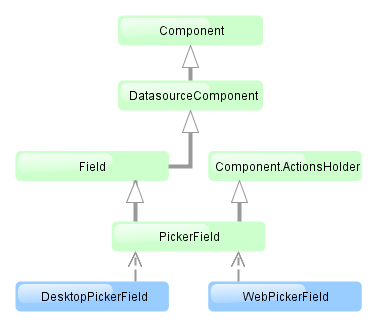
The PickerField
component is implemented for Web Client and Desktop Client.
-
As a rule,
PickerField
is used for working with reference entity attributes. It is sufficient to specify datasource and property attributes for the component:<dsContext> <datasource id="carDs" class="com.company.sample.entity.Car" view="_local"/> </dsContext> <layout> <pickerField datasource="carDs" property="colour"/>
In the example above, the screen defines
carDs
data source for aCar
entity having thecolour
attribute. In thepickerField
element, a link to a data source is specified in thedatasource
attribute, and a name of an entity attribute is set in theproperty
attribute. The entity attribute should refer to another entity, in the example above it isColour
.
-
For
PickerField
, you can define an arbitrary number of actions, displayed as buttons on the right. It can be done either in the XML descriptor using theactions
nested element, or programmatically in the controller usingaddAction()
.-
There are standard actions, defined by the
PickerField.ActionType
:lookup
,clear
,open
. They perform the selection of a related entity, clearing the field and opening the edit screen for a selected related entity, respectively. When declaring standard actions in XML, you do not have to define any attributes except the identifier. If no actions in theactions
element are defined when declaring the component, the XML loader will definelookup
andclear
actions for it. To add a default action, for example,open
, you need to define theactions
element as follows:<pickerField datasource="carDs" property="colour"> <actions> <action id="lookup"/> <action id="open"/> <action id="clear"/> </actions> </pickerField>
The
action
element does not extend but overrides a set of standard actions. Identifiers of all required actions have to be defined explicitly. The component looks as follows:Use
addLookupAction()
,addOpenAction()
andaddClearAction()
to set standard actions programmatically. If the component is defined in the XML descriptor withoutactions
nested element, it is sufficient to add missing actions:@Inject protected PickerField colourField; @Override public void init(Map<String, Object> params) { colourField.addOpenAction(); }
If the component is created in the controller, it will get no default actions and you need to explicitly add all necessary actions:
@Inject protected ComponentsFactory componentsFactory; @Override public void init(Map<String, Object> params) { PickerField colourField = componentsFactory.createComponent(PickerField.NAME); colourField.setDatasource(carDs, "colour"); colourField.addLookupAction(); colourField.addOpenAction(); colourField.addClearAction(); }
You can parameterize standard actions. The XML descriptor has limited abilities to do this: there is only
openType
attribute, in which you can specify the mode to open a selection screen (forLookupAction
) or edit screen (forOpenAction
).If you create actions programmatically, you can specify any properties of
PickerField.LookupAction
,PickerField.OpenAction
andPickerField.ClearAction
objects returned by methods of adding standard actions. For example, you can set a specific lookup screen as follows:PickerField.LookupAction lookupAction = customerField.addLookupAction(); lookupAction.setLookupScreen("customerLookupScreen");
For more information, see JavaDocs for standard actions classes.
-
Arbitrary actions in the XML descriptor are also defined in the
actions
nested element, for example:<pickerField datasource="carDs" property="colour"> <actions> <action id="lookup"/> <action id="show" icon="icons/show.png" invoke="showColour" caption=""/> </actions> </pickerField>
You can programmatically set an arbitrary action as follows:
@Inject protected PickerField colourField; @Override public void init(Map<String, Object> params) { colourField.addAction(new AbstractAction("show") { @Override public void actionPerform(Component component) { showColour(colourField.getValue()); } @Override public String getCaption() { return ""; } @Override public String getIcon() { return "icons/show.png"; } }); }
The declarative and programmatic creation of actions is described in Actions. The Action Interface section.
-
-
PickerField
can be used without binding to entities, i.e., without setting datasource and property. In this case,metaClass
attribute should be used to specify an entity type forPickerField
. For example:<pickerField id="colourField" metaClass="sample$Colour"/>
You can get an instance of a selected entity by injecting the component into a controller and invoking its
getValue()
method.WarningFor proper operation of the
PickerField
component you need either set ametaClass
attribute, or simultaneously set datasource and property attributes. -
You can use keyboard shortcuts in PickerField, see Keyboard Shortcuts for details.
- Attributes of pickerField
-
align - caption - captionProperty - datasource - description - editable - enable - height - id - metaClass - property - required - requiredMessage - stylename - visible - width
- Elements of pickerField