5.5.2.1.14. FieldGroup
FieldGroup
is intended for the joint display and editing of multiple entity attributes.
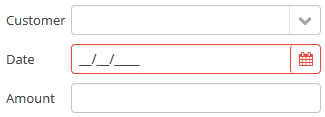
XML-name of the component: fieldGroup
The component is implemented for Web Client and Desktop Client.
Below is an example of defining a group of fields in an XML screen descriptor:
<dsContext>
<datasource id="orderDs"
class="com.sample.sales.entity.Order"
view="order-with-customer">
</datasource>
</dsContext>
<layout>
<fieldGroup id="orderFieldGroup" datasource="orderDs" width="250px">
<field property="date"/>
<field property="customer"/>
<field property="amount"/>
</fieldGroup>
</layout>
In the example above, dsContext
defines an orderDs
data source, which contains a single instance of the Order
entity. The data source is specified in the datasource
attribute of the fieldGroup
component. field
elements refer to the entity attributes that need to be displayed in the component.
Elements of fieldGroup
:
-
column
– optional element that allows you to position fields in multiple columns. For this purpose,field
elements should be placed not immediately withinfieldGroup
, but within acolumn
. For example:<fieldGroup id="orderFieldGroup" datasource="orderDs" width="100%"> <column width="250px"> <field property="num"/> <field property="date"/> <field property="amount"/> </column> <column width="400px"> <field property="customer"/> <field property="info"/> </column> </fieldGroup>
In this case, fields will be arranged in two columns; the first column will contain all fields with the width of
250px
, the second one with the width of400px
.Attributes of
column
:-
width
– specifies the field width of a column. By default, fields have the width of200px
. In this attribute, the width can be specified both in pixels and in percentage of the total horizontal width of the column.
-
flex
– a number, which indicates the degree of horizontal change in the overall size of the column relative to other columns as a result of changing the entire width offieldGroup
. For example, you can specifyflex=1
for a column, andflex=3
for another one.
-
id
– an optional column identifier, which allows you to refer to it in case of screen extension.
-
-
field
– the main component element. It defines one field of the component.Custom fields can be included in the
field
element as inline XML definition:<fieldGroup> <field id="demo"> <lookupField id="demoField" datasource="userDs" property="group"/> </field> </fieldGroup>
Attributes of
field
:-
id
– required attribute, ifproperty
is not set; otherwise it takes the same value asproperty
by default. Theid
attribute should contain an arbitrary unique identifier either of a field with theproperty
attribute set, or a programmatically defined field. In the latter case, thefield
should have the attributecustom="true"
as well (see below).
-
property
- required attribute, ifid
is not set; it should contain an entity attribute name, which is displayed in the field, for data binding.
-
caption
− allows you to specify a field caption. If not specified, an entity attribute localized name will be displayed. -
inputPrompt
- if the inputPrompt attribute is available for the component used for this field, you can set its value directly for the field.
-
visible
− allows you to hide the field together with the caption.
-
datasource
− allows you to specify a data source for the field, other than specified for the entirefieldGroup
component. Thus, attributes of different entities can be displayed in a field group. -
optionsDatasource
specifies a name of a data source, used to create a list of options. You can specify this attribute for a field connected to a reference entity attribute. By default, the selection of a related entity is made through a lookup screen. IfoptionsDatasource
is specified, you can select the related entity from a drop-down list of options. Actually, specifyingoptionsDatasource
will lead to the fact that LookupPickerField will be used in the field instead of PickerField.
-
width
− allows you to specify the field width excluding caption. By default, the field width will be200px
. The width can be specified both in pixels and in percentage of the total horizontal width of the column. To specify the width of all fields simultaneously, you can use thewidth
attribute of thecolumn
element described above.
-
custom
– if set totrue
, it means that a field identifier does not refer to an entity attribute, and a component, which is in the field, will be set programmatically usingsetComponent()
method ofFieldGroup
(see below).
-
the
generator
attribute is used for declarative creation of custom fields: you can specify the name of the method that returns a custom component for this field:<fieldGroup datasource="productDs"> <column width="250px"> <field property="description" generator="generateDescriptionField"/> </column> </fieldGroup>
public Component generateDescriptionField(Datasource datasource, String fieldId) { TextArea textArea = componentsFactory.createComponent(TextArea.class); textArea.setRows(5); textArea.setDatasource(datasource, fieldId); return textArea; }
-
link
- if set totrue
, enables displaying a link to an entity editor instead of an entity picker field (supported for Web Client only). Such behaviour may be required when the user should be able to view the related entity, but should not change the relationship.
-
linkScreen
- contains the identifier of the screen that is opened by clicking the link, enabled in thelink
attribute.
-
linkScreenOpenType
- sets the screen opening mode (THIS_TAB
,NEW_TAB
orDIALOG
).
-
linkInvoke
- contains the controller method to be invoked instead of opening the screen.
The following attributes of
field
can be applied depending on the type of the entity attribute displayed in the field:-
If you specify a value of the
mask
attribute for a text entity attribute, MaskedField with an appropriate mask will be used instead of TextField. In this case, you can also specify thevalueMode
attribute.
-
If you specify a value of the
rows
attribute for a text entity attribute, TextArea with the appropriate number of rows will be used instead of TextField. In this case, you can also specify thecols
attribute. -
For a text entity attribute, you can specify the
maxLength
attribute similarly to one described for TextField. -
For an entity attribute of the
date
ordateTime
type, you can specify thedateFormat
andresolution
for the parameterization of the DateField component used in the field. -
For an entity attribute of the
time
type, you can specify theshowSeconds
attribute for the parameterization of the TimeField component used in the field.
-
Attributes of fieldGroup
:
-
The
border
attribute can be set either tohidden
orvisible
. Default ishidden
. If set tovisible
, thefieldGroup
component is highlighted with a border. In the web implementation of the component, displaying a border is done by adding thecuba-fieldgroup-border
CSS class.
-
captionAlignment
attribute defines the position of captions relative to the fields in within theFieldGroup
. Two options are available:LEFT
andTOP
.
-
fieldFactoryBean
: declarative fields defined in the XML-descriptor are created with theFieldGroupFieldFactory
interface. In order to override this factory, use this attribute with the name of your customFieldGroupFieldFactory
implementation.For the
FieldGroup
created programmatically, use thesetFieldFactory()
method.
Methods of the FieldGroup
interface:
-
addField
enables adding fields to the FieldGroup at runtime. As a parameter it takes aFieldConfig
instance, you can also define the position of the new field by addingcolIndex
androwIndex
parameters.
-
bind()
method applied to the field aftersetDatasource()
triggers the creation of field components.
-
createField()
is used to create a FieldGroup element implementingFieldConfig
interface:fieldGroup.addField(fieldGroup.createField("newField"));
-
getComponent()
returns a visual component, which is located in a field with the specified identifier. This may be required for additional component parameterization, which is not available through XML attributes offield
described above.To obtain a reference to a field component in a screen controller, you can use injection instead of the explicit invocation of
getFieldNN("id").getComponentNN()
. To do this, use the@Named
annotation and provide an identifier offieldGroup
and a field identifier after a dot.For example, in a field selecting a related entity, you can add an action to open an instance and remove the field cleaning action as follows:
<fieldGroup id="orderFieldGroup" datasource="orderDs"> <field property="date"/> <field property="customer"/> <field property="amount"/> </fieldGroup>
@Named("orderFieldGroup.customer") protected PickerField customerField; @Override public void init(Map<String, Object> params) { customerField.addOpenAction(); customerField.removeAction(customerField.getAction(PickerField.ClearAction.NAME)); }
To use
getComponent()
or to inject field components, you need to know which component type is located in the field. The table below shows the correspondence between entity attribute types and components created for them:Entity attribute type Additional conditions Field component type Related Entity
optionsDatasource
is specifiedEnumeration (
enum
)string
mask
is specifiedrows
is specifiedboolean
date
,dateTime
time
int
,long
,double
,decimal
mask
is specifiedUUID
MaskedField with hex mask
-
removeField()
enables removing fields at runtime byid
.
-
setComponent()
method is used to set your own field view. Can be used together with thecustom="true"
attribute of thefield
element or with the field created programmatically by thecreateField()
method (see above). When used withcustom="true"
, the datasource and the property should be set up manually.The
FieldConfig
instance can be obtained withgetField()
orgetFieldNN()
method, then thesetComponent()
method is called:@Inject protected FieldGroup fieldGroup; @Inject protected ComponentsFactory componentsFactory; @Inject private Datasource<User> userDs; @Override public void init(Map<String, Object> params) { PasswordField passwordField = componentsFactory.createComponent(PasswordField.class); passwordField.setDatasource(userDs, "password"); fieldGroup.getFieldNN("password").setComponent(passwordField); }
- Attributes of fieldGroup
-
align - border - caption - captionAlignment - datasource - editable - enable - fieldFactoryBean - id - height - stylename - visible - width
- Attributes of column
- Attributes of field
-
caption - captionProperty - cols - custom - datasource - dateFormat - description - editable - enable - generator - id - link - linkInvoke - linkScreen - linkScreenOpenType - mask - maxLength - optionsDatasource - property - required - requiredMessage - resolution - rows - showSeconds - tabIndex - visible - width
- Elements of field
-
column - field - inputPrompt - validator
- API
-
addField - bind - createField - getComponent - removeField - setComponent - setFieldFactory