3.9.8.1. Uploading Files
Files from the user’s computer can be uploaded into the storage using the FileUpload and FileMultiUpload components. Usage examples are provided in this manual in the appropriate component descriptions, as well as in Loading and Displaying Images.
FileUpload
component is also available within the ready-to-use FileUploadDialog
window designed to load files in the temporary storage.
The temporary client-level storage (FileUploadingAPI
) stores temporary files in the folder defined by cuba.tempDir application property. Temporary files can remain in the folder in case of any failures. The clearTempDirectory()
method of the cuba_FileUploading
bean is invoked periodically by the scheduler defined in the cuba-web-spring.xml file.
The FileUploadDialog
window provides the base functionality for loading files into the temporary storage. It contains the drop zone for drag-and-dropping files from outside of the browser and the upload button.
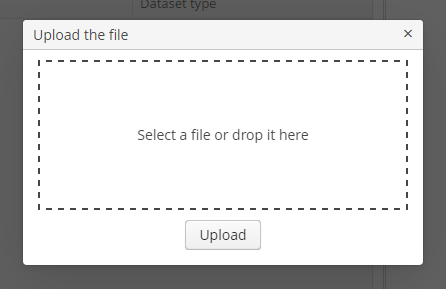
The dialog is implemented with the legacy screen API and can be used as follows:
@Inject
private Screens screens;
@Inject
private FileUploadingAPI fileUploadingAPI;
@Inject
private DataManager dataManager;
@Subscribe("showUploadDialogBtn")
protected void onShowUploadDialogBtnClick(Button.ClickEvent event) {
FileUploadDialog dialog = (FileUploadDialog) screens.create("fileUploadDialog", OpenMode.DIALOG);
dialog.addCloseWithCommitListener(() -> {
UUID fileId = dialog.getFileId();
String fileName = dialog.getFileName();
File file = fileUploadingAPI.getFile(fileId); (1)
FileDescriptor fileDescriptor = fileUploadingAPI.getFileDescriptor(fileId, fileName); (2)
try {
fileUploadingAPI.putFileIntoStorage(fileId, fileDescriptor); (3)
dataManager.commit(fileDescriptor); (4)
} catch (FileStorageException e) {
throw new RuntimeException(e);
}
});
screens.show(dialog);
}
1 | - obtaining the java.io.File object which points to the file in the file system of the Web Client block. You may need it if you want to process the file somehow instead of just putting it into the file storage. |
2 | - creating a FileDescriptor entity. |
3 | - uploading file to the file storage of the middle tier. |
4 | - saving the FileDescriptor entity. |
After successful upload, the dialog is closed with COMMIT_ACTION_ID
. In a CloseWithCommitListener
, use the getFileId()
and getFileName()
methods to get the UUID and the name of uploaded file. Then you can get the file itself or create a FileDescriptor
and upload the file to the file storage, or implement any other logic.