5.8.3.2. Initialization Using CreateAction
If the initial value of an attribute depends on the data of the invoking screen, you can use setInitialValues()
method of the CreateAction class.
Let us look at the example of two linked entities:
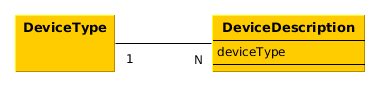
A fragment of a screen XML descriptor showing the lists of two entities simultaneously:
<dsContext>
<collectionDatasource id="typesDs"
class="com.haulmont.sample.entity.DeviceType"
view="_local">
<query>
select e from sample$DeviceType e
</query>
</collectionDatasource>
<collectionDatasource id="descriptionsDs"
class="com.haulmont.sample.entity.DeviceDescription"
view="_local">
<query>
select e from sample$DeviceDescription e where e.deviceType.id = :ds$typesDs
</query>
</collectionDatasource>
</dsContext>
<layout>
...
<table id="typeTable">
<actions>
<action id="create"/>
<action id="edit"/>
<action id="remove"/>
</actions>
<columns>
<column id="name"/>
</columns>
<rows datasource="typesDs"/>
</table>
...
<table id="descriptionTable">
<actions>
<action id="create"/>
<action id="edit"/>
<action id="remove"/>
</actions>
<columns>
<column id="description"/>
</columns>
<rows datasource="descriptionsDs"/>
</table>
</split>
</layout>
The screen controller:
public class DeviceTypeBrowse extends AbstractLookup {
@Inject
private CollectionDatasource<DeviceType, UUID> typesDs;
@Named("descriptionTable.create")
private CreateAction descrCreateAction;
@Override
public void init(Map<String, Object> params) {
typesDs.addItemChangeListener(event -> {
descrCreateAction.setInitialValues(Collections.<String, Object>singletonMap("deviceType", event.getItem()));
});
}
}
A listener is added in the controller for selected record change event in the typesDs
datasource. When the selected record is changed, the system invokes the action’s setInitialValues()
method and submits a map with one element whose key is the attribute name (deviceType
) and value (the selected instance of DeviceType
). Thus, during the execution of CreateAction
, the deviceType
attribute of the new DeviceDescription
instance will contain the instance of the DeviceType
that was selected in the table.