4.2.1.1. Base Entity Classes
The base entity classes and interfaces are described in detail in this section.
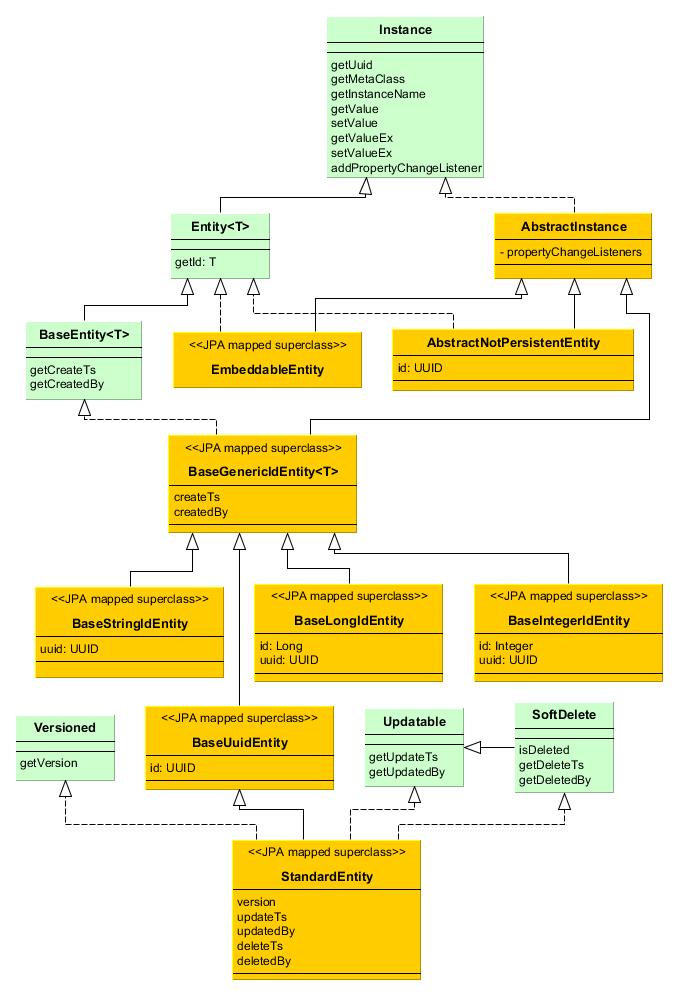
-
Instance
– declares the basic methods for working with objects of application domain:-
getting the global unique identifier (UUID) of the entity
-
getting references to the object meta-class
-
generating the instance name
-
reading/writing attribute values by name
-
adding listeners receiving notifications about attribute changes
-
-
Entity
– extendsInstance
with entity identifier (which is not necessarily equal to the UUID); at the same timeEntity
does not define the type of the identifier leaving this option to descendants. -
AbstractInstance
– implements the logic of working with attribute change listeners.WarningAbstractInstance
stores the listeners inWeakReference
, and if there are no external references to the added listener, it will be immediately destroyed by garbage collector. Normally, attribute change listeners are visual components and UI datasources that are always referenced by other objects, so there is no problem with listeners dropout. However, if a listener is created by application code and no objects refer to it in a natural way, it is necessary to save it in a certain object field apart from just adding it toInstance
. -
AbstractNotPersistentEntity
– base class of non-persistent entities withUUID
identifier. -
BaseEntity
– base class of persistent entities; declares methods for working with information about when and by whom the entity instance was created in the database. -
BaseGenericIdEntity
– implementsBaseEntity
with added JPA annotations without specifying the type of the identifier (i.e. the primary key) of the entity. -
BaseUuidEntity
– extendsBaseGenericIdEntity
and sets theid
identification attribute of theUUID
type. -
BaseLongIdEntity
– extendsBaseGenericIdEntity
and sets theid
identification attribute of theLong
type. -
BaseIntegerIdEntity
– extendsBaseGenericIdEntity
and sets theid
identification attribute of theInteger
type. -
BaseStringIdEntity
– extendsBaseGenericIdEntity
and sets only the type of the identifier -String
. A specific entity class, extended fromBaseStringIdEntity
, must have aString
-type identifier attribute with the@Id
JPA annotation. -
Versioned
– interface for entities supporting optimistic locking. -
Updatable
– interface for entities which require to keep the information about when and by whom the instance was last changed. -
SoftDelete
– interface for entities supporting soft deletion. -
StandardEntity
– the most commonly used base class of persistent entities that implements the interfaces given above.
When creating entity classes it is recommended to choose a base class according to the following rules:
-
If an entity is not stored in the database, inherit it from
AbstractNotPersistentEntity
. -
If an entity is embedded, inherit it from
EmbeddableEntity
. -
If an entity is only created in DB, never changes and does not need soft deletion, inherit it from
BaseUuidEntity
. -
If an entity behaves in a standard way: changes in the database, requires optimistic locking and soft deletion − inherit it from
StandardEntity
. -
Otherwise inherit the entity from
BaseUuidEntity
and implementVersioned
,Updatable
,SoftDelete
interfaces if required. -
For some entities, it is desirable to use integer or string primary keys. In this case, inherit the entity from
BaseLongIdEntity
,BaseIntegerIdEntity
orBaseStringIdEntity
instead ofBaseUuidEntity
.