6.1. Task Execution Sample
This sample demonstrates the following:
-
How to programmatically create process actors on process start using the
ProcActionsFrame
-
How to pass process variables to the process instance using the
ProcActionsFrame
-
How to get and modify standard process actions created by the
ProcActionsFrame
(e.g. change "Start process" button caption) -
How to start a process programmatically without the
ProcActionsFrame
-
How to automatically update the
processState
field each time the process moves further using theActivitiEventListener
The sample uses the Task execution - 1 process model:
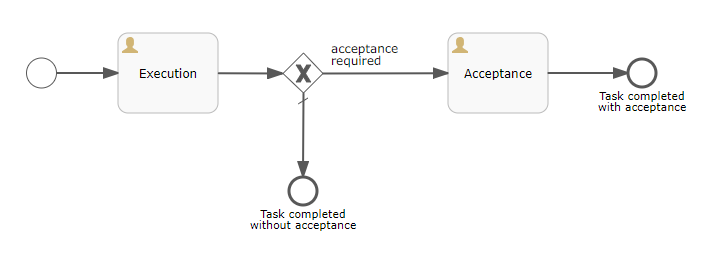
To test the sample, use the Application - Tasks screen.
In this example, we don’t use the StandardProcForm
to assign process actors. We do it with the help of the before start process predicate of the ProcActionsFrame
. See the setBeforeStartProcessPredicate()
method usage in the TaskEdit.java
See the setStartProcessActionProcessVariablesSupplier()
usage in the TaskEdit.java as an example of how to pass process variables at process start using the ProcActionsFrame
. The acceptanceRequired
process variable will be used by one of process gateways to decide whether the task must be accepted by the initiator or the process must be completed.
The changeStartProcessBtnCaption()
demonstrates that you can get and modify process actions generated by the ProcActionsFrame
. In this method the standard button caption "Start process" is replaced by the custom one.
The startProcessProgrammatically()
method demonstrates how to start a new process instance without the ProcActionsFrame
.
public void startProcessProgrammatically() {
if (commit()) {
//The ProcInstanceDetails object is used for describing a ProcInstance to be created with its proc actors
BpmEntitiesService.ProcInstanceDetails procInstanceDetails = new BpmEntitiesService.ProcInstanceDetails(PROCESS_CODE)
.addProcActor("initiator", getItem().getInitiator())
.addProcActor("executor", getItem().getExecutor())
.setEntity(getItem());
//The created ProcInstance will have two proc actors. None of the entities is persisted yet.
ProcInstance procInstance = bpmEntitiesService.createProcInstance(procInstanceDetails);
//A map with process variables that must be passed to the Activiti process instance when it is started.
//This variable is used in the model to make a decision for one of gateways.
HashMap<String, Object> processVariables = new HashMap<>();
processVariables.put("acceptanceRequired", getItem().getAcceptanceRequired());
//Starts the process. The "startProcess" method automatically persists the passed procInstance with its actors
processRuntimeService.startProcess(procInstance, "Process started programmatically", processVariables);
showNotification(getMessage("processStarted"));
//refresh the procActionsFrame to display complete tasks buttons (if a process task appears for the current
//user after the process is started)
initProcActionsFrame();
}
}
The UpdateProcessStateListener.java is an implementation of the org.activiti.engine.delegate.event.ActivitiEventListener
. This listener is registered as a process-level listener. It does the following: each time a new process step is reached, the processState
field of the related com.company.bpmsamples.entity.Task
entity is updated with the current process step name.
That’s how process-level event listeners configuration looks in the process model.
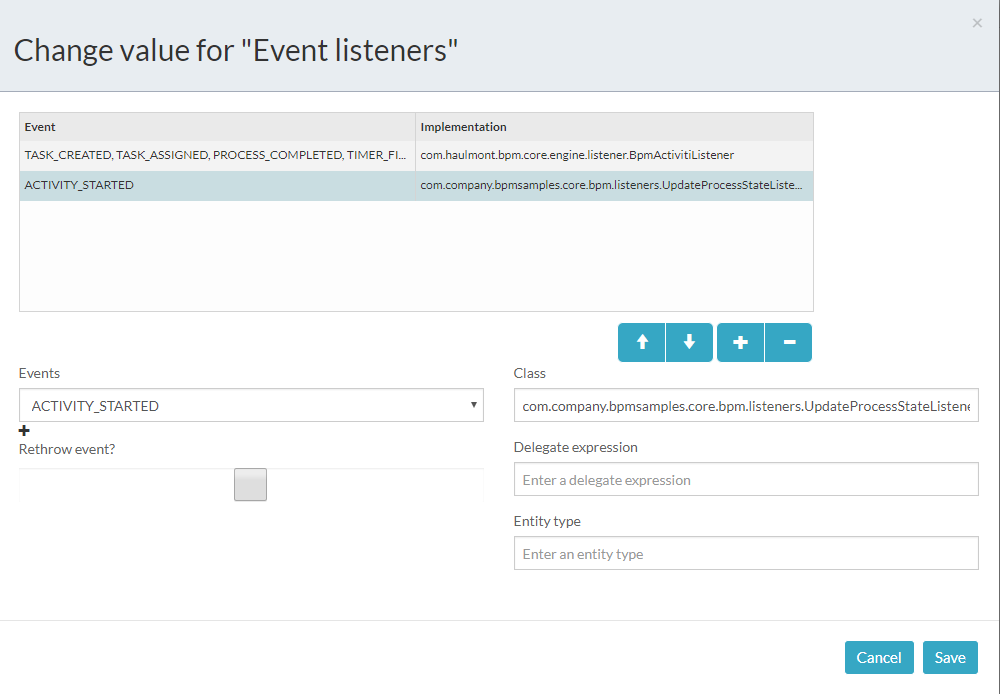
To open this window click somewhere in the modeler, click the Show advanced properties link and then go with the Event listeners property.
See the comments in the source code at GitHub for details.
The Task entity editor XML descriptor: task-edit.xml
The Task entity editor Java controller: TaskEdit.java
Activiti event listener that updates processState: UpdateProcessStateListener.java